Automating Email Forwarding with AWS Lambda, SNS, and SES
- Published on
- Authors
- Name
- Binh Bui
- @bvbinh
Automating Email Forwarding with AWS Lambda, SNS, and SES
Introduction
In today's digital age, managing emails efficiently is crucial for businesses and individuals alike. Automating email forwarding can save time and ensure that important messages are never missed. In this blog post, we will explore how to set up an AWS Lambda function to automatically forward emails received via AWS SNS (Simple Notification Service) to a specified email address using AWS SES (Simple Email Service). This guide is designed for beginners and will walk you through the entire process step-by-step.
Prerequisites
Before we dive into the setup, make sure you have the following:
- An AWS account.
- Basic knowledge of AWS services like Lambda, SNS, and SES.
- Python installed on your local machine.
Setting Up the Environment
Step 1: Create an SNS Topic
First, we need to create an SNS topic that will receive the email content in Base64 format.
- Log in to your AWS Management Console.
- Navigate to the SNS service.
- Click on "Create topic".
- Choose a name for your topic and create it.
Step 2: Create a Lambda Function
Next, we will create a Lambda function that will process the emails and forward them to the specified recipients.
- Navigate to the Lambda service in your AWS Management Console.
- Click on "Create function".
- Choose "Author from scratch".
- Give your function a name and choose Python as the runtime.
- Click on "Create function".
Step 3: Configure the Lambda Function
Now, we need to configure the Lambda function to process the emails.
- In the Lambda function's code editor, paste the following code:
import json
import boto3
import base64
import os
from email import message_from_bytes
from email.policy import default
def lambda_handler(event, context):
try:
print(f"Received event: {json.dumps(event)}")
sns_message = event['Records'][0]['Sns']['Message']
sns_data = json.loads(sns_message)
email_content_base64 = sns_data['content']
try:
email_content = base64.b64decode(email_content_base64)
except Exception as decode_error:
print(f"Error decoding base64 content: {decode_error}")
return {'statusCode': 400, 'body': json.dumps('Invalid base64 encoding')}
try:
msg = message_from_bytes(email_content, policy=default)
from_email = msg.get('From', 'Unknown Sender')
to_email = msg.get('To', 'Unknown Recipient')
subject = msg.get('Subject', 'No Subject')
body = None
if msg.is_multipart():
for part in msg.walk():
content_type = part.get_content_type()
content_disposition = str(part.get("Content-Disposition", ""))
if content_type == "text/plain" and "attachment" not in content_disposition:
charset = part.get_content_charset() or 'utf-8'
body = part.get_payload(decode=True).decode(charset, errors='replace')
break
else:
charset = msg.get_content_charset() or 'utf-8'
body = msg.get_payload(decode=True).decode(charset, errors='replace')
if body is None:
body = "No plain text content found."
except Exception as parse_error:
print(f"Error parsing email: {parse_error}")
return {'statusCode': 400, 'body': json.dumps('Error parsing email content')}
print(f"From: {from_email}")
print(f"To: {to_email}")
print(f"Subject: {subject}")
print(f"Body: {body}")
ses_client = boto3.client('ses')
email_to = os.environ.get('EMAIL_TO', '').split(',')
email_from = os.environ.get('EMAIL_FROM', '')
if not email_to or not email_from:
print("No recipient email configured")
return {'statusCode': 400, 'body': json.dumps('No recipient email configured')}
body = f"{body}\n\n---\n\nThis email was forwarded by Lambda function {context.function_name}"
response = ses_client.send_email(
Source=email_from,
Destination={'ToAddresses': email_to},
Message={
'Subject': {'Data': f"Fwd: {subject}"},
'Body': {'Text': {'Data': f"From: {from_email}\nTo: {to_email}\n\n{body}"}}
}
)
print(f"SES response: {response}")
return {'statusCode': 200, 'body': json.dumps('Email content extracted and forwarded successfully')}
except boto3.exceptions.Boto3Error as ses_error:
print(f"SES Error: {ses_error}")
return {'statusCode': 502, 'body': json.dumps('Error sending email via SES')}
except Exception as e:
print(f"Unhandled error: {e}")
return {'statusCode': 500, 'body': json.dumps('Internal server error')}
- Set the environment variables
EMAIL_TO
andEMAIL_FROM
with the recipient email address and sender email address, respectively.
Step 4: Subscribe Lambda to SNS Topic
- Navigate back to the SNS topic you created.
- Click on "Create subscription".
- Choose "AWS Lambda" as the protocol.
- Select the Lambda function you created.
- Click on "Create subscription".
Step 5: Test the Setup
To test the setup, send an email to the email address configured in the SES mail receipt rule. The email should be forwarded to the SNS topic, which will trigger the Lambda function. The Lambda function will process the email and forward it to the recipients specified in the EMAIL_TO environment variable.
Conclusion
By following this guide, you have successfully set up an AWS Lambda function to automatically forward emails received via SNS to a specified email address using SES. This automation can save you time and ensure that important messages are never missed. Feel free to explore more AWS services and enhance your email processing workflows.
For more details and to access the code, visit the GitHub repository.
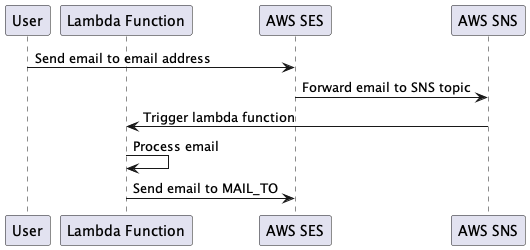
Thank you for reading! If you have any questions or feedback, please leave a comment below.